Serialization/Deserialization Series: XML
How do you transform your XML and JSON data into objects that are validatable? Today, we cover how to take only an XML document and create objects to validate against your business rules.
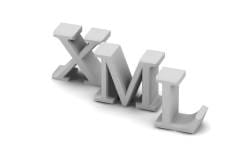
Serialization/Deserialization Series
I've recently posted a quick tip on how to Debug XmlSerializer Errors if you are interested.
Back in 2006, I worked for a company that had a project where they had certain requirements of taking an XML document and making them into reusable objects, but allowing an easier way to manipulate them in a system.
When I was given this task, I read about a technique that takes XML, or any data format, and have a "reader" create the nodes while reading in the document.
The technique is called serialization and deserialization.
Serialization is the process of taking an object and converting it into a stream of bytes to be read or used later in a database, object, or file.
As you can probably guess, deserialization is the opposite. It's the process of taking that stream and converting it into an object that's usable in another part of a system. An excellent example is a web.config.
Nine years later, I'm working with serialization and deserialization again.
Nothing like coming around full circle, huh?
Creating The Objects From XML
Let's start with a sample XML file. I created a simple dashboard.xml from my CMS dashboard.
<?xml version="1.0" encoding="utf-8" ?> <dashboard> <tabs> <tab title="Home"> <widget id="15" title="Weather"> </widget> <widget id="19" title="RSS Feed"> </widget> </tab> <tab title="Sports"> <widget id="21" title="Scores"> </widget> </tab> </tabs> </dashboard>
Now that we have our XML, how do we create our objects?
Simple.
I refer back to my earlier post on the "Ludicrous Speed" coding series. Paste the XML as classes into a plain Class1.cs file. Of course, make sure your project is targeted to a .NET 4.5 Framework for this to work properly.
Once your code is pasted, your classes will be generated in the editor. The type of classes generated may look a little different. Start renaming them to classes that are more meaningful instead of DashboardTabsTabWidget.
Some tips when using these classes:
- Use either ReSharper's Safe Renaming or Visual Studio's Rename (Refactor, then Rename) to make sure you don't lose any classes that have a different name. It'll keep your sanity. Trust me.
- If you have a different name for your XML elements, use an XmlElement attribute on your classes. For example, if you want your Widget class to be called Component, do a safe-rename of the widget class to Component and place
[XmlElement("Widget")]
on the top of your Component class. - If you have a large XML file, I would recommend using ReSharper's Move Types Into Matching Files. This will make changes to your XML classes more manageable in the long run.
- I have removed the partials from the classes since I will be modifying them and not regen-ing them any time soon.
Now that you have your classes split out, we need to make our serializer.
Let's Get Deserial!
Since we already have our XML, we might as well create our object by deserializing the XML into our object.
To create a deserializer, we need to include the System.Xml.Serialization
namespace.
var xml = @"<?xml version=""1.0"" encoding=""utf-8"" ?> <dashboard> <tabs> <tab title=""Home""> <widget id=""15"" title=""Weather""></widget> <widget id=""19"" title=""RSS Feed""></widget> </tab> <tab title=""Sports""> <widget id=""21"" title=""Scores""></widget> </tab> </tabs> </dashboard>"; var stream = xml.ToStream(); StreamReader reader = new StreamReader(stream); XmlSerializer serializer = new XmlSerializer(typeof(dashboard)); var dashboard = (dashboard) serializer.Deserialize(reader); return dashboard;
NOTE: The ToStream() is taken from the post called 10 Extremely Useful .NET Extension Methods. It takes a string and converts it into a MemoryStream.
After running the little test, we have a fully-hydrated dashboard object ready for processing.
Push it back out!
Now that we have our dashboard object, let's go the other way and serialize the object into XML.
The serialization process is just as easy.
var sb = new StringBuilder(); var ns = new XmlSerializerNamespaces(); ns.Add("", ""); using (var reader = XmlWriter.Create(sb)) { serializer.Serialize(reader, dashboard, ns); reader.Close(); }
NOTE: The ToStringContents() is another .NET extension method that I reference. It's in the Stream section, but it's not called ToStringContents(). It's just called ToString().
Once you have your XML, you can store it in a file, database, or object.
Conclusion
Today, we went over how to quickly add classes that mirror our XML document and also demonstrated how we can serialize and deserialize those objects for later use in other systems.
Later this week, we'll go over the serializing and deserializing of JSON objects as well as cover a way to validate all of our objects.
Serialization/Deserialization Series
Serialization/Deserialization Series