Quick Tip: Debug XmlSerializer Errors
Serialization and deserialization can be rough because it can be a black box. Today, I show you how to find out what's happening during the serialization/deserialization process.
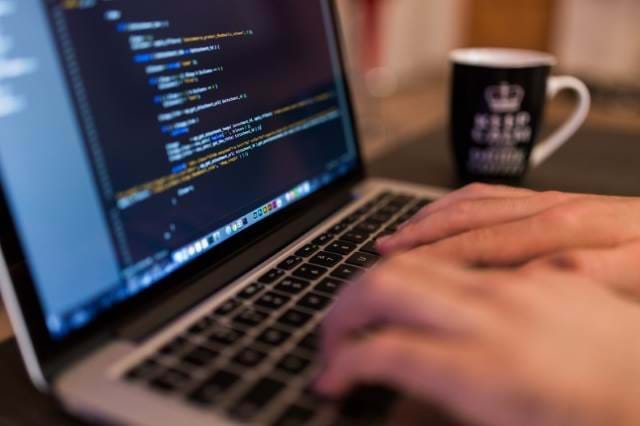
One of the hardest things to detect is whether serialization or deserialization is working when you use XmlSerializer or a DataContractSerializer.
In my Serialization/Deserialization Series, I use the XmlSerializer and found life to be easy.
However, there are times when you want to use an XmlSerializer over a DataContractSerializer. Here are some of the differences:
DataContractSerializer
- It's faster than XmlSerializer (10% to be technical about it).
- Used specifically for WCF services to serialize/deserialize to/from Json or XML.
- Serializes properties and fields (IMPORTANT: if XML, they MUST be in alphabetical order).
XmlSerializer
- Only used for serializing/deserializing XML
- Allows for customizable serializing
- Only public properties are serialized.
The reason I bring these differences up is because XmlSerializer is a little more customizable allowing more flexibility when serializing or deserializing your objects.
You can add XML attributes to your code making a property an attribute or element where as DataContractSerializer is a little bit harder to control.
Opening the Black Box
Way back in 2005, there wasn't a DataContractSerializer so for our specific project, we had to use an XmlSerializer.
I was recently experiencing difficulties as to why objects weren't deserializing and forgot about how to check to see what was happening under the covers.
The XmlSerializer class has events detailing the deserialization process as it reads your XML.
These events occur when the serializer can't determine how to deserialize the object based on the XML you feed it.
Is it a problem with an Attributes, Node, Element, or Unreferenced Object?
var serializer = new XmlSerializer(typeof(Vendor)); serializer.UnknownAttribute += (sender, args) => { System.Xml.XmlAttribute attr = args.Attr; Console.WriteLine($"Unknown attribute {attr.Name}=\'{attr.Value}\'"); }; serializer.UnknownNode += (sender, args) => { Console.WriteLine($"Unknown Node:{args.Name}\t{args.Text}"); }; serializer.UnknownElement += (sender, args) => Console.WriteLine("Unknown Element:" + args.Element.Name + "\t" + args.Element.InnerXml); serializer.UnreferencedObject += (sender, args) => Console.WriteLine("Unreferenced Object:" + args.UnreferencedId + "\t" + args.UnreferencedObject.ToString());
Of course, you execute this before you perform your deserialize.
Conclusion
The team I work with have wrestled with some XmlSerializer and DataContractSerializer issues in the past and these events provide clues on how to better structure your XML.
I'm pretty sure there are also some legacy applications out there still using XmlSerializers and thought this would be a great quick tip on debugging XML through an XmlSerializer.
How do you debug your XmlSerializer errors? Do you still use XmlSerializer? Are you using DataContractSerializer? Post your tips below and let's discuss.