Serialization/Deserialization Series: JSON
What happens when a client asks for JSON for an AJAX service instead of XML? Today, I discuss the way to return your data as JSON instead of XML.
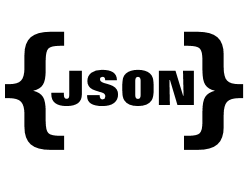
Serialization/Deserialization Series
- XML
- Json
- Custom Serialization
I've also recently posted a quick tip on how to Debug XmlSerializer Errors if you are interested.
In the last post, I discussed the way to serialize and deserialize an object based on XML.
We took an existing XML file and used Visual Studio's Paste XML as Classes to generate our XML objects so we can read in XML to create our objects (deserialize) or write out a modified XML document from our objects (serialize).
While XML is easy, JSON (JavaScript Object Notation) is becoming the better way to send data across the wire because of the natural and minimal structure to reduce the verbosity of an XML document.
Hey JSON!
In the Edit menu, there is also a Paste JSON as Classes option. While we could use that and make more classes, we can reuse our existing class to serialize JSON as well as our XML.
To serialize an object into JSON, you need to include a JavaScriptSerializer which can be found in the System.Web.Script.Serialization
namespace.
Based on the Dashboard example we used in the previous post, we would pass the dashboard object into the meat-grinder...errr...JavaScript serializer. :-)
var x = new JavaScriptSerializer(); var json = x.Serialize(dashboard);
The json variable contains the following:
{ "tabs" : [ { "title" : "Home", "widget" : [ { "id" : 15, "title" : "Weather" }, { "id" : 19, "title" : "RSS Feed" } ] }, { "title" : "Sports", "widget" : [ { "id" : 21, "title" : "Scores" } ] } ] }
No Node!
Wait a minute...what happened to our root node? Unfortunately, it excludes the root node and serializes the object's data inside the object. It doesn't include the root object. Unfortunately, this is the nature of the beast. It's how the Serializer works.
If you want to include the root node, place the object in a wrapper like so:
public class JsonContainer { public dashboard Dashboard { get; set; } public JsonContainer(dashboard dashboard) { Dashboard = dashboard; } }
And then in your code, you would pass in the JsonContainer object.
var container = new JsonContainer(dashboard); var x = new JavaScriptSerializer(); var json = x.Serialize(container);
This is probably the easiest way to the root node included.
Your new JSON will look like this:
{ "dashboard" : { "tabs" : [ { "title" : "Home", "widget" : [ { "id" : 15, "title" : "Weather" }, { "id" : 19, "title" : "RSS Feed" } ] }, { "title" : "Sports", "widget" : [ { "id" : 21, "title" : "Scores" } ] } ] } }
Conclusion
Since we covered the XML serialization/deserialization procedure before, I felt it fitting to discuss the JSON serialization process and gotchas in this post even though this is a short post.
The JavaScript Serializer is another way to take an object structure using simple objects with native types and easily convert them into JSON objects.
Did I miss something? Post a comment below to continue the discussion.
Serialization/Deserialization Series
- XML
- Json
- Custom Serialization