Quick Tip: Building an ASP.NET MVC RSS ActionResult
For those looking to create their own RSS feed, today I talk about creating an RSS feed from scratch.
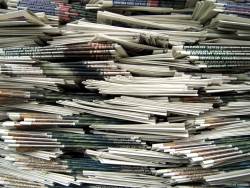
RSS feeds have been around for a long time and, to be honest, not many people know what an RSS feed can do. I discussed it back in 2006 in a RSS beginner's guide on my site to educate my readers.
Today, I am still using an RSS reader (Feedly). Before Feedly, I was using Google Reader (R.I.P.). And before THAT, I was using FeedDemon (yeah, I'm showing my age).
To most uneducated users of the Internet, I consider an RSS Reader to be the same as reading the newspaper. As a matter of fact, a judge even ruled that surfing the Internet was like using a telephone or reading a newspaper.
Nothing wrong with that. You are getting your news in a simple digest or summary for the day...you just receive a constant flood of news 24/7. Of course,you can even mark a story to view it later if you want.
But enough about what RSS feeds are...we're going to build one today using an custom ActionResult.
What's an ActionResult?
An ActionResult signifies what can be returned from a Controller. The most standard ASP.NET MVC ActionResult is returned at the bottom of a method when you see:
return View();
The View() is disguised as a ViewResult(). Since this is so commonly used in Controllers, there is a method called View in the controller that creates a new instance of a ViewResult() and returns it to the View (HTML).
There are other ActionResults like FileResult, ContentResults, and even ExcelActionResults which we built previously.
What we're going to do today is create our own RssActionResult by inheriting from an ActionResult.
The code for the RssActionResult is as follows:
Mvc\ActionResults\RssActionResult.cs
public class RssActionResult : ActionResult { public SyndicationFeed Feed { get; set; } public override void ExecuteResult(ControllerContext context) { context.HttpContext.Response.ContentType = "application/rss+xml"; var rssFormatter = new Rss20FeedFormatter(Feed); using (var writer = XmlWriter.Create(context.HttpContext.Response.Output)) { rssFormatter.WriteTo(writer); } } }
Anti-climatic, isn't it?
If you wanted to use this for a top 10 Latest Posts RSS feed, you would create the following in a controller:
public ActionResult Posts() { IEnumerable<Post> posts = _postRepository.GetAllByDate(10); var feed = new SyndicationFeed("Latest Posts","DanylkoWeb RSS Feed", new Uri("https://www.danylkoweb.com/RSS"), Guid.NewGuid().ToString(), DateTime.Now); var items = new List<SyndicationItem>(); foreach (Post bp in posts) { string postUrl = String.Format("blog\\BlogEntry-{0}", bp.Id); var item = new SyndicationItem(bp.Title, bp.Description, new Uri(postUrl), bp.Id, bp.PublishOn.Value); items.Add(item); } feed.Items = items; return new RssActionResult {Feed = feed}; }
The Syndication classes are located in the System.ServiceModel.Syndication
namespace.
The SyndicationItem is a collection contained in the SyndicationFeed class. All we are doing is populating the SyndicationItem with the Post properties that are related to each other.
In this example, we used Blog Posts, but you can use other types of data to store in an RSS feed. You can use events, top menu items for the day, or top-rated products on your site.
Now that you know how to create an RSS feed, you can create any type of RSS feed you want to keep your audience up to date as to what is happening on your site or with your products.