Part 1: Transform XML/JSON into Classes
In this first post, we show how to take existing data and immediately convert them into classes to use in your code.
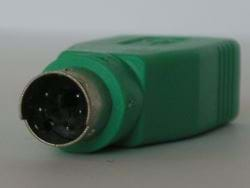
There are many file formats out there and most everyone (I think) uses databases, but what happens when a brand new project starts, you already have an existing database stabilized, and a new vendor wants to send you their data in an XML format...or a CSV...or a JSON format to import into the system.
Wonderful!
When you receive this format, I'm sure a lot of developers have thoughts of working late at night trying to assemble the code to read the file format flawlessly.
This first post from the "Ludicrous Speed" series talks about the various ways to immediately convert, import, and export your data from XML/JSON/CSV into workable classes.
First, we need a data file. Since I'm heading to Codemash v2.0.1.5 in January, we will use the Sessions and Speakers API found at Codemash 2015 API.
XML
I'll just get this one right out of the way since it's a standard and easy file format.
There are two ways to convert XML into classes:
- XSD.EXE (XML Schema Definition Tool)
The XML Schema Definition Tool has been around since the 1.1 .NET days and comes with Visual Studio (any version).
The tool is located in your Visual Studio 201x Programs folder (Start/All Programs/Visual Studio 2013/Visual Studio Tools or Start/All Programs/Microsoft Visual Studio 201x/Visual Studio Tools).
The simplest way to use this tool is to type in XSD.exe to see the parameters and make sure you have access to the XML file. To use it, type the following:XSD.EXE speakers.xml /c
There will be a class generated with the same name as the XML file except the .xml will be replaced with a .cs extension. - Paste Special
This has been in Visual Studio since 2012 and it has by far saved my butt a number of times.
However, this feature has some requirements attached to it. Your project needs to be targeted towards the 4.5 .NET framework for this to work. Otherwise, the "Paste Special..." option will not be available on the Edit menu.
This is probably THE quickest and easiest way to convert your data formats into code that I have ever seen.
- Find the XML that you need to convert to code. In our case with Codemash Speakers, go to https://cmprod-speakers.azurewebsites.net/api/speakersdata, view the source, select all, and copy it to the clipboard.
- Create a new class in your project. It's OK if it's called Class1.cs. :-)
- Under the Edit dropdown, select Paste Special and select Paste XML as Classes.
- BOOM! Done! I renamed some of the classes for readability and made some auto-properties. Here is the one class generated and modified.
namespace Codemash.Classes { /// <remarks/> [System.Xml.Serialization.XmlTypeAttribute(AnonymousType = true, Namespace = "http://schemas.datacontract.org/2004/07/CodeMash.Speakers.Web.Models")] public class CodemashSpeaker { /// <remarks/> public string Biography { get; set; } /// <remarks/> [System.Xml.Serialization.XmlElementAttribute(IsNullable = true)] public object BlogUrl { get; set; } /// <remarks/> public string FirstName { get; set; } /// <remarks/> [System.Xml.Serialization.XmlElementAttribute(IsNullable = true)] public object GitHubLink { get; set; } /// <remarks/> public string GravatarUrl { get; set; } /// <remarks/> public string Id { get; set; } /// <remarks/> public string LastName { get; set; } /// <remarks/> [System.Xml.Serialization.XmlElementAttribute(IsNullable = true)] public object LinkedInProfile { get; set; } /// <remarks/> [System.Xml.Serialization.XmlElementAttribute(IsNullable = true)] public object TwitterLink { get; set; } } }
public override IEnumerable<CodemashSession> GetRecords(string xmlData) { var serializer = new XmlSerializer(typeof(ArrayOfPublicSessionDataModel)); var buffer = Encoding.UTF8.GetBytes(xmlData); using (var stream = new MemoryStream(buffer)) { var sessions = (ArrayOfPublicSessionDataModel)serializer.Deserialize(stream); return sessions.Sessions; } }
JSON
If you didn't see this coming based on the previous XML entry, go back and read it. ;-)
The easiest way to convert your JSON into classes is to perform the same steps above, but with a JSON file, click Paste Special, and select Paste JSON as classes.
Now, use either the JavaScriptSerializer or the Newtonsoft's JsonSerializer.
There are also online Json converters. The only one I know of that I recommend is json2csharp.
There is also an open-source application called JsonClassGenerator.
CSV
For converting CSV files into C# classes, this can be a chore, but it is definitely easier as a single file instead of a hierarchical structure like JSON or XML.
If you don't include header information, it'll be a tough conversion process because you won't have descriptive class members (field1, field2, field3, etc). Jeff Brand has a good idea for creating a simple CSVPosition Attribute to your code.
The CSV format have a couple of complications.
- Does the CSV format have quotes around strings?
- Are there commas in a string that would mess up the import if comma-delimited (i.e. 1234 Main Road, Suite 123)?
I think I may have to revisit this later and maybe come up with a process to speed up the importing on a whim based on a CSV and present it to everyone.
Stay tuned!
Overall
When you need to create classes based on data in a hurry, these techniques can make your life a lot easier.
Series: How to take your ASP.NET MVC development to ludicrous speeds.
- Transforming XML/JSON/CSV into Classes
- Use pre-built applications
- T4 (Text Template Transformation Toolkit)
- ReSharper/SQL Complete
- ORM (Object Relationship Management)
What techniques do you use for converting data into classes? Post your comments below.