What are the differences between ASP.NET MVC and ASP.NET WebForms?
Since 2002, Web Forms was always the method for developing Microsoft Web Applicatons...until MVC came along. Today, I talk about the differences between Web Forms and MVC.
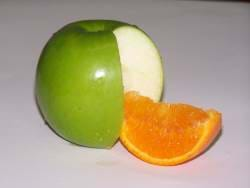
Before ASP.NET, I was using Classic ASP and using Delphi 2/3 to build ISAPI dynamically linked libraries, or DLLs. The searching capability on the companies web site was my first achievement in the corporate world using a custom ISAPI library.
Then, along came ASP.NET in 2002 and changed the landscape. C# was relatively new and Visual Basic developers started the transition of moving from Visual Basic to VB.NET and experiencing a compiled state of web development. Some stayed with VB 6 (no comment).
That's when Web Forms were introduced with ASP.NET.
Ever since that day, I still have issues with Web Forms and it's quirky-ness. Always having to check and make sure you include an IsPostBack as the first line in your Page_Load, understanding how to keep your bloated ViewState variable under control, minimizing the payload back to the client without using too many web controls, and so on and so on and so on.
The web development process didn't feel natural to me.
Flash forward to 5 years later (2007), ASP.NET MVC CTP was introduced.
Again, the landscape changed.
Not only did it change, but it moved more towards a standard way of development.
Some developers screamed that ASP.NET MVC was a step backwards and that we were moving back to the Classic ASP days again, but in my eyes, it was absolutely the right step for Microsoft.
It felt like a natural web development process once again.
For a new developer to experience the web through the eyes of Microsoft, I would absolutely recommend ASP.NET MVC. But since 2002, there are thousands, if not millions, of Web Form applications out there that have a legacy. Some of the Web Forms coding is...let's just say...different.
Terminology
Before we proceed to talk about the differences between WebForms and MVC, we need to know the difference between certain terms on the Microsoft stack of technologies. In the past, I've heard a couple of developers mixing and matching certain terms that weren't related to each other at all.
- .NET Framework - A technology introduced in 2002 which includes the ability to create executables, web applications, and services using C# (pronounced see-sharp), Visual Basic, and F# (wikipedia)
- ASP.NET - An open source, server-side web application framework which is a subset of the .NET Framework that focuses specifically on building web application, web sites, and web services.
- ASP.NET Web Forms - (2002 - current) A proprietary technique developed by Microsoft to manage state and form data across multiple pages. The innate ability of the web are state-less pages where Microsoft created stateful pages by creating the Web Forms technique.
- ASP.NET MVC - (2008 - current) An open source web application framework that implements a Model-View-Controller design pattern.
With that cleared up, let's go over the differences between ASP.NET Web Forms and MVC.
Differences Between ASP.NET Web Forms and ASP.NET MVC
Even though these technologies have been around for 5+ years, they each have their advantages and disadvantages.
- Web Forms has ViewState, MVC does not.
This has become the bane of my existence. As I mentioned above, ViewState is a page-level state management mechanism. If you have a number of server-side web controls on a page, your ViewState will become extremely large.
Let me tell you a story of how ViewState can be so...unnatural.
I worked at one company where I wasn't getting any data back from a submitted form. After talking to the security specialists and the networking team, I found out that my requests were being blocked by the company firewall.
The reason? The firewall was blocking the request because there was a form trying to perform a 2MB+ postback and the firewall thought it was blocking an attack from a hacker.
Another reason is that your HTML SHOULD be small to send back to the client, not bloated with a huge 1MB+ ViewState variable. Let's see that pass the mobile-friendly test. :-)
MVC does not have a ViewState. It uses model/ViewModels to pass back and forth between the Views. - Web Forms has a Code-Behind Model where MVC just has models.
Code-Behind is a way to attach C# or VB code to a web page and act on their specific actions when they occur on the page. When you compile a Web Form that contains code-behind, it becomes part of the assembly (or DLL) to make the site functional.
With MVC, you pass in data that was already processed and is being delivered to the View which should have no logic or processing included in it as well. - Web Forms has Web Controls where MVC does not.
There are a lot of developers who were complaining that MVC didn't have any server-side controls. This is because they became spoiled with the Web Forms way of doing things on a page. Controls were already available to them and they used them. Eventually, they started building their own custom server controls.
While Web Forms had a large number of server-controls, MVC kept the lean-and-mean approach to granular HTML. After the dust settled, developers started to understand the direction with MVC and leveraged JavaScript where they could build their own custom controls or even use a third-party library like Bootstrap for their UI. - Web Forms has State-aware components where MVC uses more of a template approach
This is why it's hard to convert a Web Forms application over to an MVC application.
In Web Forms, developers place controls on a web page and are able to manipulate those controls on the server in the code-behind. The server-side controls are even aware of their state when ViewState is turned on.
With MVC, you aren't working with controls, you are passing data to be used in a dumb View. The Razor syntax in a View is extremely powerful. If you want an objects property dropped into the HTML HEAD tag, you place a @model.Title right in the header and you're done. - Where Web Forms doesn't have the separation of concerns, MVC is adamant about it!
I can't tell you how many times I've seen developers create a Web Form, attach code to a control, and then place the business logic inside the code-behind instead of being handled or processed by a business object. How is that business logic going to be used in another application when it's attached to a UI control?
MVC's philosophy with business logic is "Thin Controllers, Fat Models" (Hence, my article about the skinniest controller) meaning that the models will contain all of your business logic while your controllers should return a model to the view.
This forces you to think about how your models should already have "processed data" and how the ViewModel will strictly be a car to transport your processed models to your destination which is the View. - Web Forms and MVC can use Sessions, but I would recommend against it.
Along with ViewState, sessions are another bane of my existence. Sessions provide state in a state-less web. What happens when that state is out of sync? Don't tell me it won't be because it will. Been there, done that!
Developers placing state (or worse, user controls) inside of a session is just asking for trouble.
In MVC, there are a number of ways of passing data back and forth to the View. I mention a couple in a post called ASP.NET MVC Views: How to Pass Data To Views.
As I mentioned before, I'm glad I'm not the only one who feels this way about Sessions. - Web Forms has IsPostBack where MVC has a GET and...well...a POST
New Web Form developers (and some seasoned) sometimes forget to include an "if (IsPostBack) return;" statement in their Page_Load event of their page. This may look strange to some veteran web developers coming over from PHP or other web language.
The whole idea of Web Forms is when you are requesting a page, it's considered a GET (visiting the page for the first time) and the page initializes.
The term IsPostback is coined when a form is submitted, or POST-ed. So if I visit a page, the IsPostBack lets me through and performs the initialization of the page. When you submit a form, the Page_Load detects that it's a Postback and kicks you out of the Page_Load immediately because it should defer to the event that triggered the submit.
MVC has the standard HTTP Protocol standards of GET, POST, DELETE, and redirects. When you click a submit button, that form (and data) gets submitted to the controller and the controller handles the processing of it.
Again, the MVC way feels more natural. - Web Forms is not testable-friendly, MVC was built around tests.
Out of the box, web form developers have a problem.
Unless they were disciplined with their business objects and code-behind forms, it may take a rewrite to get most of the business logic into a testable state.
With MVC, you can build tests against the controller, routes, action results...against just about any hooks in the ASP.NET MVC Framework.
- Web Forms has One. Big. Form. MVC can have multiple forms on a page.
Speaking of unnatural, you can imagine how hard it is to go to a website that has a JavaScript service that contains an HTML form and they suggest you copy-and-paste this sample code into your ASP.NET Web Forms page.
Won't work. Why?
If you do a View Source on a Web Forms web site, you'll notice that right after the BODY tag in your HTML, there is a Form tag that encompasses the entire page...INSIDE A FORM!
This is where the gears in my head locked up the first time I experienced this. How kooky is this?
MVC allows you to have multiple forms on a page.
End of story.
Conclusion
After experiencing both technologies in a ten-year period, I am very thankful that ASP.NET MVC arrived when it did. I immediately snatched it up to experience a smoother way to develop.
As I said before, it was more natural. It "feels" like the web now. POST, GET, DELETE, Redirect.
With vNext arriving soon, there are a couple of items that some developers may want to pay particular attention to in this post by Stephen Walther about the Top 10 Changes In ASP.NET 5 and MVC 6.
The two that stand out to me are No More Web Forms and No More Visual Basic.
It's what I consider a move towards standards instead of using proprietary technology.
You can't make standards without a "stand."
Did I miss something in my differences between Web Forms and MVC? Post a comment below.