Quick Tip: Unit Testing with ASP.NET Core
With ASP.NET Core, unit testing has changed. In today's post, I show my readers how to create a quick unit test for a .NET Core project or class library.
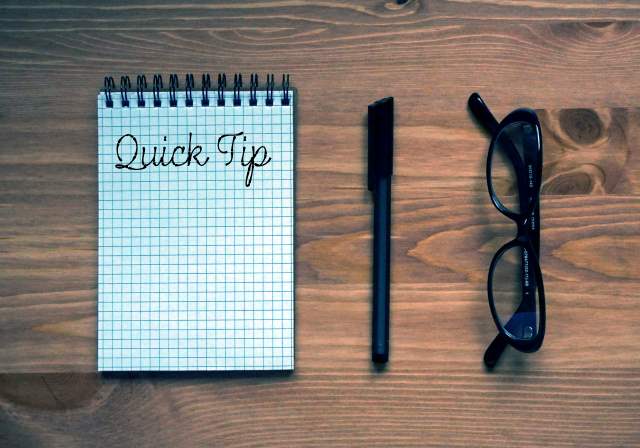
Unit testing is now a standard in development and provides certain checks and balances to confirm our code behaves as expected.
With .NET Core (and 1.1 released), we now experience something a little different from before.
This quick tip today shows you how to add a unit test to an existing .NET Core project.
First Steps
Once you have your project loaded, it can be a simple .NET Core project or it can be a Class Library.
Class Library
Once you have your class library loaded, you need to create a package for your unit test since we are now a NuGet community, right?
- Build your Class Library.
- Open a command-prompt to your class library's project (or you can also use Mad's Web Extension Pack 2015 extension called Open Command Line to Alt-Space to the directory immediately).
- Type:
dotnet pack
(This will create a NuGet package and place it into your assembly folder).
You are now ready to create your Unit Test project.
Project
The difference between the class library and project is the project doesn't need to be packaged so you can ignore these first steps if you have a project.
Creating the Unit Test
With .NET Core, the key is to get away from Unit Test "Projects" and create Unit Test Class Libraries.
To get started:
- Create a .NET Core Class Library.
- Modify your project.json file to look like this:
{ "version": "1.0.0-*", "testRunner": "mstest", "dependencies": { "dotnet-test-mstest": "1.0.1-preview", "MSTest.TestFramework": "1.0.0-preview", "NETStandard.Library": "1.6.1" }, "frameworks": { "netcoreapp1.0": { "imports": [ "dnxcore50", "portable-net45+win8" ], "dependencies": { } } } }
- Finally, add your class library (or project) to your dependencies and use the version of "1.0.0-*" to get started (in bold).
"dependencies": { "dotnet-test-mstest": "1.0.1-preview", "MSTest.TestFramework": "1.0.0-preview", "MyClassLibrary": "1.0.0-*", "System.Linq.Queryable": "4.3.0", "Microsoft.NETCore.App": "1.1.0", "NETStandard.Library": "1.6.1" },
You are all set to run your unit tests.
I kept creating a Unit Test Project and didn't put 2 and 2 together to find out unit testing Core applications required a Class Library, not a project.
Luckily, I found a post about MSTest supporting the .NET Core Framework while I was starting to convert a project from ASP.NET MVC to ASP.NET MVC Core.
I'm posting this quick tip to assist anyone else who may need it in the future...
...and that includes me.