Quick Tip: How to use multiple submit buttons on one FORM
Most submitted forms have one button to submit the data. What happens when you have 2 or more buttons? This post will cover a technique on how to act on numerous button functions in one form tag.
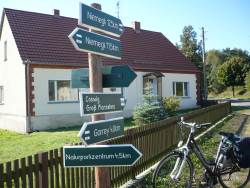
Before the ASP.NET MVC framework was introduced in 2007, buttons on a WebForm were easier to trace using click events. You double-click on the button and pow, you have an event attached to it or you add it through code-behind.
Now, let's switch over to ASP.NET MVC. Forms are a little different now. There aren't any fancy names for them, no fancy technology names, they're just regular Internet forms as defined by the W3C, passing data through a POST.
However, with the regular forms, there isn't a simple way to place two or more buttons on a page and have each button act out a different action.
So my question is how do you have more than one button on a form execute different code when the form is submitted?
Back to Basics
While working on a project back in 2009, there was a WebForm that had four buttons on it. Of course, ViewState was running on the page (ughh!) and each one was easy to code because of the event handler. However, the client wanted to convert it to an MVC project.
When forms are submitted, they are passed using the name of the control and the value in a name/value pair. The ASP.NET MVC framework makes this easy for us to check using the POST method of your Action in your controller.
Time to Refactor!
Let's look at the HTML.
@using (Html.BeginForm("Create", "Blog", FormMethod.Post)) { @Html.AntiForgeryToken() <button type="submit" id="publish" name="command" value="publish" class="btn btn-primary">Publish</button> <button type="submit" id="draft" name="command" value="draft" class="btn btn-default">Save Draft</button> }
What is with the name on each button? It's the same?
Well, that's the secret. The name is the command you want to detect when POST-ing back and the value is the action you want to check when the button is pressed.
So what does the MVC code look like?
public ActionResult Create(CreateViewModel model, string command) { var approved = (command == "publish"); . . . }
All that needs to be done is check the command coming in and act on it.
Conclusion
Your final solution may be different, but here are the rules for passing multiple submit buttons to your forms:
- Each button has to have a unique name for each button (i.e. name="command")
- Each button needs a unique value that they can check (i.e. value="test1")
- On your POST Action in your Controller, use the unique name you used on your HTML button as a parameter, or create a model binder.
If you do create a model binder, it may seem like more code, but if you have other forms that perform multiple actions (like an email form), it can be reused and contained inside your BaseViewModel. Remember, DRY. :-)
Post your comments and questions below.