ASP.NET MVC Routing Examples
Today, I'll show you how to create a number of different routes to make your site a little more SEO-Friendly for the search engines.
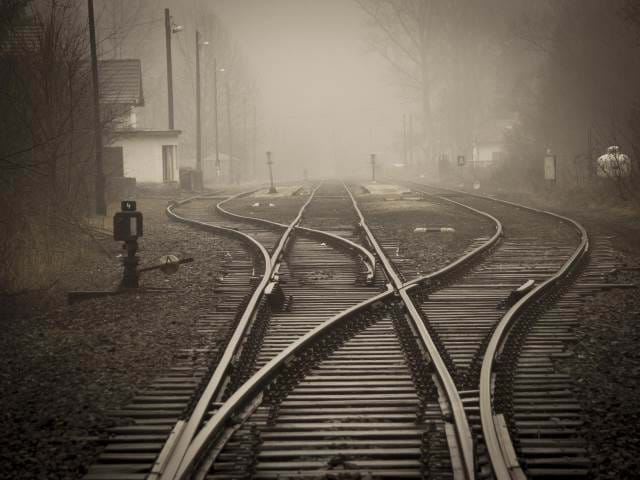
Routing is one of the fundamental concepts for ASP.NET MVC web applications.
However, routing can get confusing as your application starts to grow. You can't keep adding more and more routes to accommodate your site structure.
Your site structure is kind of important. Routing ties into:
- How people see your site
- How search engines see your site.
It's important enough to mention your routing is the index of your site. Any ASP.NET MVC URL goes through the routing engine.
It not only sends the users to the page, but provides a certain level of professionalism when they first visit your site and see a human-friendly URL.
How important is it? Rand Fishkin from Moz mentioned how important it is to have SEO-friendly URLs on your site.
If you are still unsure on how to define a URL structure for your site that's SEO-friendly, I would also recommend Andy Baran's post from SEOptimer.com titled Everything You Wanted to Know About URLs (And Best Practices for their Optimization).
Even Google wrote up content guidelines on their Google Search Console support site mentioning the simpler your site's URL structure, the better.
Why?
If a user clicks on a search result pointing to a page on your site, they won't arrive through the main page. They could arrive on a deep page you haven't even touched in two years.
When they get there, you want to make sure they can look at the URL and know exactly where they're located on your site.
You may need visual cues or indicators to let them know where they were dropped off.
These visual cues could include breadcrumbs, a shallow navigation menu, or even buttons to lead them to something (like a conversion).
Routing Best Practices
Learning ASP.NET MVC was a trial when it first came out and I didn't have any idea about routing.
I had routes going every-which-way where I didn't know what route called which controller action ("So that's where that went!")
After I write this post, I'll add these details under the Routing section of the ASP.NET MVC Best Practices and Guidelines, but for now, I'll describe them here.
Keep Your Routes Simple
Your URLs should give a solid description of what the intended value the web page gives to a user before they open the page.
Take this URL for example:
http://mycookingsite.com/?tag=casserole&id=234231
Hmm...I know we are heading to a cooking site with a specific tag of casserole, but what's the id?
Is it a tag id or a post id?
Your URLs shouldn't be cryptic and inform the user what page they're heading to on your site.
Keep Your Routes To A Minimum
Your ASP.NET MVC routes are stored in your RouteConfig.cs file in the App_Start folder.
You want to keep these routes to a minimum as much as you can.
I've experienced one application containing over 30 routes.
30 Routes!
These routes had the actual controller name hard-coded in the route definition where the controller pattern ({controller}) would've work just fine by itself.
Once optimized, these routes were distilled down to five routes.
Shallow, not Deep
The number of folders makes a difference on a site.
As an example, which URL would you prefer?
http://mycookingsite.com/blog/fred-rotars/casseroles/eggs/holidays
or
http://mycookingsite.com/blog/holiday-egg-casserole
It makes sense to use the second URL as opposed to the first because search engines see the second URL as something simple where you have a blog with a post describing how to make a holiday egg casserole.
The smaller the URL, the better.
A general rule-of-thumb would be to verbally repeat the URL. If someone can type it in from memory, it's a pretty good indicator you have a solid URL structure.
Create a URL Reference Table
It's always good to have an "at-a-glance" view of your URL site structure.
It also makes sense to write out the URLs of how you see them once your site is finished.
I like to place these into my RouteConfig.cs file and list out all of the URLs with the controller and action it executes.
// URL Controller Action Id Pattern
// /Blog/this-is-my-blog-title-QW Blog Index QW {controller}/{title}-{id}
This gives me an idea of how my URLs are used throughout the site.
ASP.NET MVC Routing Examples
With the best practices behind us, we can now examine some routing examples.
Example 1
URL: /tag/mobile
Route:
routes.MapRoute( name: "TagRoute", url: "Tag/{id}", defaults: new { controller = "Tag", action = "Index", id = UrlParameter.Optional } );
Description:
This URL is meant to hard-route a tag to a specific action. Behind the scenes, we can say we want this pattern to go to the Index page with the id passed into it.
Look up the tag name in the database, page the data, and return all of the posts pertaining to the mobile tag.
Why didn't we leave the {controller} in the URL?
If we did, the site would always default to this TagRoute and not even get to the DefaultRoute so we have to add a special Url to catch all of our tag URL calls.
Example 2
URL: /Blog/This-is-a-test-post-title-UU
Route:
routes.MapRoute( name: "BlogPage", url: "Blog/{title}-{id}", defaults: new { controller = "Blog", action = "Index", title = String.Empty } );
Description:
Ok, I cheated. I used this example above and it's the URL structure for DanylkoWeb.
This URL could very easily been /Blog/this-is-a-test-post-title/UU.
Again, because this is a custom URL structure, we need to make MVC aware of any page with the Blog in the URL is sent to the Blog controller and Index action.
Notice the title? IF it's not provided, it will be blank. We only need the id to retrieve our blog post.
The controller action would look like this:
public ActionResult Index(string title, string id)
If title was included (which it should be), it would be passed in along with the id. ASP.NET MVC Routing is smart enough to parse them out and pass them through to the Index Action.
Example 3
URL: /Blog/2017/07/21/this-is-a-test-post-title
Route:
routes.MapRoute("BlogArchive", "Blog/{year}/{month}/{day}/{title}", new { controller = "Blog", action = "List", month = "1", day = "1" }, new { year = @"\d{2}|\d{4}", month = @"\d{1,2}", day = @"\d{1,2}" } );
Description:
This is more of a WordPress URL and uses constraints to confirm we have a four digit year, two digit month, and a one- or two-digit day.
The controller action would look like this:
public ActionResult Index(string year, string month, string day, string title)
Any curly brackets in the URL is passed into the controller's designated action.
You decide whether to act on them or not.
Example 4
Url: /ebooks
Route:
routes.MapRoute( name: "LandingPage", url: "{id}", defaults: new { controller = "LandingPage", action = "Index" } );
Description:
If you want a landing page for specific opt-ins, you can make a single word URL by removing everything but the id in the URL.
The id default was removed to force an id. The controller (LandingPageController) and action (Index) will always receive an id of a word (in this case, eBooks).
Whatever word is passed into the LandingPageController/Index action, you need to perform a lookup to retrieve how you want the page displayed for "ebooks", "newsletter", or whatever word triggers a conversion.
Conclusion
These routes are the most common next to the /controller/action/id syntax.
Make your site structure as consistent as possible because most of the search engines will reward you with a well-structured site.
Is there a specific route you use for your site? Is it similar to one of these routes? Post your comment below and let's discuss.