ASP.NET MVC Optimization Series: Introduction and Setup
In this optimization series, we discuss how to setup and prepare for building a regular web app to make it look, act, and feel like a native app.
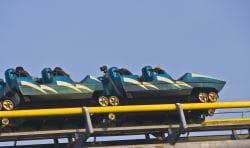
I'm always up for a challenge. On Monday, I mentioned why I was moving towards a web-based approach for mobile as opposed to native or hybrid.
If you have an optimized web page and you pulled out all the stops to use every optimization technique, you could make the webpage responsive and perform like a native or hybrid.
For this entire series, we'll explore how to take a simple web application and optimize it to pass as a native or hybrid application.
In the first part of this series, we'll setup the environment and requirements for our project.
Overview (or What the heck are we building?)
I've been going to Codemash since 2011 and it's definitely been eye-opening every time I go.
One of the apps that everyone seems to use is a native app to manage their sessions they want to attend at the conference.
So I thought, why not push the limits of an ASP.NET MVC with a Codemash event manager web app using HTML5, CSS, and JavaScript? The only thing we have to work with is the data feeds from this year's conference (2015).
The data we're working with is located on an Azure web site. The feeds are listed below:
- Sessions - https://cmprod-speakers.azurewebsites.net/api/SessionsData
- Speakers - https://cmprod-speakers.azurewebsites.net/api/SpeakersData
That's it. That's what we have to work with in our web app.
Also, I want to make sure everyone knows the angle I'm coming from when designing this web app. For me to pull this off, I need some goals for this project to be a success. These are the goals I'm focusing on:
- Speed - Since the smart phones have the native speed, we need to optimize the web app as much as humanly possible. Again...we need to come up with as many speed optimizations as we can to pull this off.
- "Native" UI - We want to make this experiment as believable as possible so we need a convincing UI to show that it's just as clean and sexy as a native app on a smartphone.
To accomplish these goals, our approach to building this web application will focus on a number of techniques for optimizing the app.
- Server-side optimizations
For the server-side, we want to make sure that we aren't making multiple calls to our data when our data is already available to us and implement application caching so the client-side doesn't have to wait for it. - Client-side optimizations
Since we want our web app to be as fast as possible, we'll need to focus on local storage, sprite generation, caching, and do as much online management before we go offline. - Perception
If there is a web call we need to make or some timely process that has to happen, we need to notify the user that there is something going on instead of leaving them hanging wondering what's going on. This is the balance that we need to maintain when it comes to network latency vs. "did the app lock up?"
With that said, let's setup the project.
Creating the Project
I created a fresh ASP.NET MVC project with no authentication or WebAPI.
Once the project is generated, I modified the code to match my style of MVC apps.
Here is what I did.
- Configuration Folder - I created this folder to contain any configuration settings for the web app (i.e. access to web.config).
- Interfaces Folder - Any interfaces necessary.
- Models Folder - Contains the models from our data feeds.
- Repository and Unit Of Work Folder - Yes, yes, I'm including my data layer in my project. We could pull it out into it's own "Data Layer" project, but I decided this demo will focus more on optimization as opposed to a full-blown 3-tier application for a simple event manager.
- ViewModel Folder - Contains all of our ViewModels for the app.
- ViewModelBuilder Folder - Contains our ViewModelFactory and ViewModelBuilders to return to our Views (Ref: The Skinniest ASP.NET MVC Controllers You've Ever Seen)
Along with the folder structure, I added some additional packages to the project using NuGet.
- StructureMap (install-package StructureMap.MVC5) - I know in the past I've been using Ninject for my reflection purposes, but recently, a couple of people (and posts) have pointed out some performance issues with Ninject (A, B, C). Since one of my goals for this series is speed and reflection is a core component I'm using, I think I'll use StructureMap.
- Bootstrap (install-package bootstrap) - I need the responsiveness in a CSS library and there isn't a CSS Framework out there to handle it better than Bootstrap.
- JASNY (install-package jasny-bootstrap) - Since I mentioned JASNY as an awesome Twitter Bootstrap enhancement library, I've been itching to try out their OffCanvas feature. Now's my chance.
- Font Awesome (install-package fontawesome) - Instead of using the standard glyph icons that come with Bootstrap, this library allows multiple icon sizes, overlap of icons, and other variations that the glyph icons doesn't provide.
I'm sure we'll be adding more to our project as we move forward, but for now, these are the essential libraries for our project.
Conclusion
As we go through this series discussing why I've chosen each path, I'll show you how to turn a standard ASP.NET MVC web application into a snazzy, sexy mobile web app that acts like a native app.
In the next post of the series, we'll setup our models, focus on retrieving our data using the server and find out how to optimize the calls for our client.
Is there another library that you think would be a great fit for a mobile app? Post your comment below.