Advanced Basics: Using Transforms In Your ASP.NET Project, Part 1
With your web application, configurations are extremely important. Today, I cover configuration files in ASP.NET and why they're important.
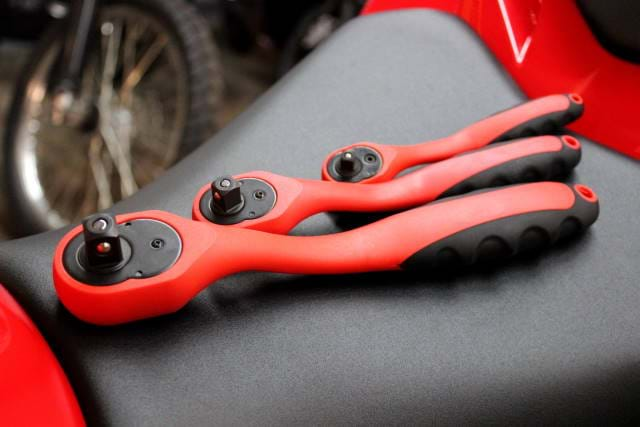
When I first started working ASP.NET web projects, I wanted to know what web.config files were and all of the different types added to a project.
I experienced them when I started working with ASP.NET back in 2002. Since everyone was so new and didn't know what "environments" were, I saw them as glorified .INI files in XML format (for you Windows 9x users).
However, with the new DevOps movement, these files are now extremely important (which I'll get into later).
The reason for the post this week is I heard some developers say while the web.config
file was important, the web.xxx.config
files were not necessary.
JUMPIN' JIMINEY!
Let's look at ASP.NET projects first.
ASP.NET Projects - web.config
When you create a brand new ASP.NET project, you automatically get a web.config
with grouped web.debug.config
and web.release.config
files placed underneath the web.config
.
This is where your settings for your application go.
Web.Config
- ALL of your settings for the application reside.Web.Debug.Config
- This is optional (you COULD have all in the web.config by default), but place your changes in here for it to work in a DEBUG environment (usually DEV)Web.Release.Config
- These changes are meant for a published release.
A sample web.config would look like this:
<?xml version="1.0" encoding="utf-8"?> <!-- For more information on how to configure your ASP.NET application, please visit https://go.microsoft.com/fwlink/?LinkId=301880 --> <configuration> <appSettings> <add key="webpages:Version" value="3.0.0.0"/> <add key="webpages:Enabled" value="false"/> <add key="ClientValidationEnabled" value="true"/> <add key="UnobtrusiveJavaScriptEnabled" value="true"/> </appSettings> <connectionStrings> <add name="MovieDBContext" connectionString="Data Source=localhost;Initial Catalog=aspnet-MvcMovie;Integrated Security=SSPI" providerName="System.Data.SqlClient" /> </connectionStrings> <system.web> <compilation debug="true" targetFramework="4.7.2"/> <httpRuntime targetFramework="4.7.2"/> </system.web> </configuration>
In Visual Studio, there is a configuration manager for building your project at the top. By default, the setting is on debug.
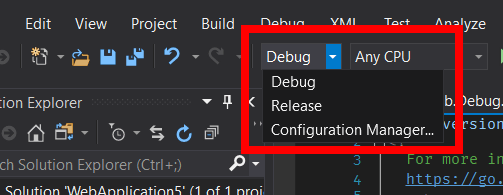
At the bottom of the dropdown, you can manage additional environments as well. You can easily add a QA, Staging, or Production setting. In this example, I added a QA setting.
After you add an additional environment, right-click the web.config
file in Visual Studio to create a new config.
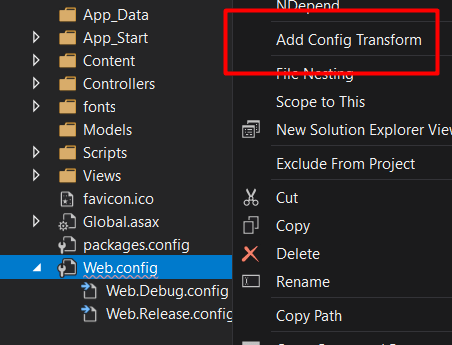
This create a new web.config
for each environment not available.
What Do You Place in Each File?
When you build your project in Visual Studio, MSBuild takes your current environment (Debug, Release, or whatever's selected in your dropdown) and transforms your web.xxx.config
settings into a new web.config
.
If you're config files are built properly, you can preview what it will look like when built.
Right-click on any file under the web.config and select "Preview Transform." You'll get a comparison of what your Web.Config looks like on the left and what the transformed Web.Config looks like.
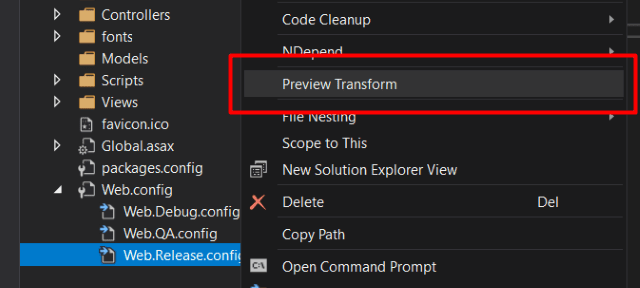
For example, in every web.config, it's best to remove the debug attribute from the compilation tag so debugging information isn't included with your deployment. This makes your web application faster.
Let's say our web.config
from above is all we need and we want to modify the connectionstring
tag and remove the debug
attribute.
Our Web.Release.Config would look like this:
<?xml version="1.0"?>
<!-- For more information on using Web.config transformation visit https://go.microsoft.com/fwlink/?LinkId=301874 -->
<configuration xmlns:xdt="http://schemas.microsoft.com/XML-Document-Transform"> <connectionStrings> <add name="MovieDBContext" connectionString="Data Source=ReleaseSQLServer;Initial Catalog=MyReleaseDB;Integrated Security=True" xdt:Transform="SetAttributes" xdt:Locator="Match(name)"/> </connectionStrings> <system.web> <compilation xdt:Transform="RemoveAttributes(debug)" /> </system.web> </configuration>
If you look at the configuration, you'll notice at the end of the connection string, we want to issue a transform on attributes and we want to match by name.
In the xdt:Locator, we want to match by name. The name in the web.config is MovieDBContext. It'll match by name and replace the attributes when transformed.
If you are looking more transformation commands, refer to the Microsoft Docs regarding XSLT Transformations.
Why are Configuration Files important?
These configuration files are important for three reasons:
- When you check code in, it downloads all of the source code from a particular location and compiles the code on a build server creating artifacts to be deployed to whatever server you choose. These configuration (
web.config
) files are important when you build your project. This gives us a way to specify which build to use for a particular environment. - It allows you to test your code locally with different environment settings for local debugging.
- These configuration settings are a centralized place for configuration settings so the DevOps team can add them to your Azure DevOps Pipeline.
Conclusion
In part one, I explained how web configurations were used in ASP.NET web projects and why they're important.
In part two, we'll dig into ASP.NET Core and how the appsettings.json are used in the project.
Do you have any tips on how to optimize your web.configs? Have you used them before? Do you use them at all? Post your comments and let's discuss.