Using Faker and NBuilder To Generate Massive Data for your Unit Tests
What do you do when you need a large amount of test data for your unit tests? In today's post, I show you how to create dummy data using NBuilder and Faker.
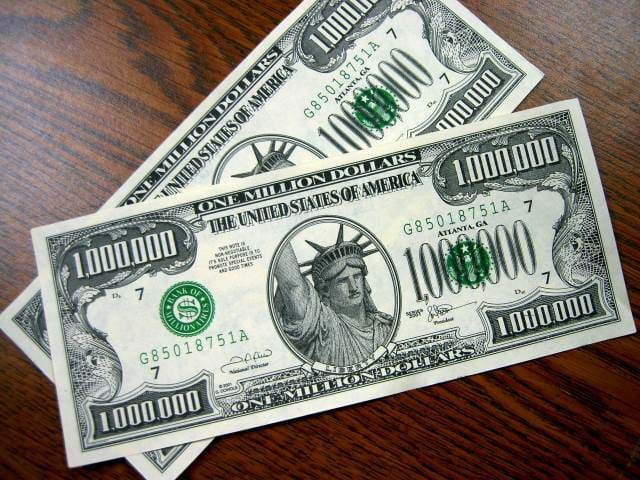
One of the hardest things in coding is naming things.
When writing unit tests, you need some test data. Sometimes even names of companies or people simulating production-style data...complete with commas and apostrophes.
Back in July of last year, I mentioned the fastest way to mock a database for unit testing, but everyone doesn't need that much depth of unit testing.
In smaller cases of unit testing, wouldn't it be nice to generate a list of 15 records with various types of values for a specific data type?
That's where NBuilder and Faker.Net enters the picture.
Getting Started
For your unit tests, download NBuilder or Faker from NuGet.
For NBuilder,
install-package NBuilder
For Faker,
install-package Faker.Net
Once you've added those libraries, you can get started immediately.
Faker.Net
Faker.Net is a library from Ben Smith and Ollie Riches that creates a random set of dummy data using static methods.
var companies = new List<string>(); var count = 100; for (var i = 0; i < count; i++) { companies.Add(Company.Name()); }
If you need 100 company names, call the static Company.Name() to create a fake company name.
Faker also allows you to create addresses, phone numbers, internet addresses, lorum ipsum text, and yes, even a name generator.
NBuilder
NBuilder is a way to create data using simple fluent syntax.
Each method provides an easy way to build your dummy data.
Following the same example from above using NBuilder instead, the code would look something like this:
var companyList = Builder<Company> .CreateListOfSize(100) .TheFirst(20).With(e => e.Title = "My Company Name") .TheNext(40).With(e=> e.Title = "Company Name that is too long.") .Build();
Now that you created your data, you can move this code into a setup method in your unit tests and test that particular data.
Some of the features in NBuilder include building a hierarchy of objects, "operable" extensions, and excellent configurability.
Which One To Use?
I know what you're thinking. Which one is better for unit testing?
Honestly, I think it's best to use both.
Use Faker for the data while using NBuilder to build the structure.
For example,
var companyList = Builder<Company> .CreateListOfSize(100) .TheFirst(50).With(e => e.Title = Faker.Company.Name()) .TheNext(50).With(e=> e.Title = Faker.Name.FullName()) .Build();
I decided to throw the Name.FullName() in there for unit testing a name instead of a company.
Conclusion
It's hard to come up with companies, names, and text to help you unit test, but Faker and NBuilder assists with all tasks including structure and, of course, naming things.
Is there another library out there to help with populating data for unit tests? Post your comments below.